|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
Quests::Q&A This is the quest support section |

03-14-2014, 12:04 AM
|
Hill Giant
|
|
Join Date: Jun 2005
Posts: 105
|
|
Augmentor Quest
Are there currently any quest commands that would augment items for players? So let's say Player A gives an NPC item and an augment, the NPC would return the item with the augment socketed in?
|

03-14-2014, 01:28 AM
|
Administrator
|
|
Join Date: May 2013
Location: United States
Posts: 1,595
|
|
You may use $client->SummonItem(), it has the following paramaters: item_id, charges, attune, aug1, aug2, aug3, aug4, aug5
I use this for some storage code I wrote that allows you to store items with augments and withdraw them.
Here's an example. Although, you could just use $item1, $item2, $item3, and $item4 if you want them to be able to put anything in anything.
Code:
sub EVENT_ITEM
{
if(plugin::check_handin(\%itemcount, 1001 => 1, 1002 => 1))
{
$client->SummonItem(1001, 1, 0, 1002);
}
}
|

03-14-2014, 09:48 AM
|
Hill Giant
|
|
Join Date: Jun 2005
Posts: 105
|
|
Quote:
Originally Posted by Kingly_Krab
You may use $client->SummonItem(), it has the following paramaters: item_id, charges, attune, aug1, aug2, aug3, aug4, aug5
I use this for some storage code I wrote that allows you to store items with augments and withdraw them.
Here's an example. Although, you could just use $item1, $item2, $item3, and $item4 if you want them to be able to put anything in anything.
Code:
sub EVENT_ITEM
{
if(plugin::check_handin(\%itemcount, 1001 => 1, 1002 => 1))
{
$client->SummonItem(1001, 1, 0, 1002);
}
}
|
This is excellent, thanks! I guess the more complicated next step would be to send in a check to make sure the augment would actually fit in the augment slot or is there any chance the summonitem code would do that automatically?
|
 |
|
 |

03-14-2014, 05:13 PM
|
Administrator
|
|
Join Date: May 2013
Location: United States
Posts: 1,595
|
|
I'd say $client->GetItemStat(), but it doesn't have augment slots according to this. Yeah, the code confirming it doesn't have augment slots is below.
Code:
uint32 Mob::GetItemStat(uint32 itemid, const char *identifier)
{
const ItemInst* inst = database.CreateItem(itemid);
if (!inst)
return 0;
const Item_Struct* item = inst->GetItem();
if (!item)
return 0;
if (!identifier)
return 0;
uint32 stat = 0;
std::string id = identifier;
for(int i = 0; i < id.length(); ++i)
{
id[i] = tolower(id[i]);
}
if (id == "itemclass")
stat = uint32(item->ItemClass);
if (id == "id")
stat = uint32(item->ID);
if (id == "weight")
stat = uint32(item->Weight);
if (id == "norent")
stat = uint32(item->NoRent);
if (id == "nodrop")
stat = uint32(item->NoDrop);
if (id == "size")
stat = uint32(item->Size);
if (id == "slots")
stat = uint32(item->Slots);
if (id == "price")
stat = uint32(item->Price);
if (id == "icon")
stat = uint32(item->Icon);
if (id == "loregroup")
stat = uint32(item->LoreGroup);
if (id == "loreflag")
stat = uint32(item->LoreFlag);
if (id == "pendingloreflag")
stat = uint32(item->PendingLoreFlag);
if (id == "artifactflag")
stat = uint32(item->ArtifactFlag);
if (id == "summonedflag")
stat = uint32(item->SummonedFlag);
if (id == "fvnodrop")
stat = uint32(item->FVNoDrop);
if (id == "favor")
stat = uint32(item->Favor);
if (id == "guildfavor")
stat = uint32(item->GuildFavor);
if (id == "pointtype")
stat = uint32(item->PointType);
if (id == "bagtype")
stat = uint32(item->BagType);
if (id == "bagslots")
stat = uint32(item->BagSlots);
if (id == "bagsize")
stat = uint32(item->BagSize);
if (id == "bagwr")
stat = uint32(item->BagWR);
if (id == "benefitflag")
stat = uint32(item->BenefitFlag);
if (id == "tradeskills")
stat = uint32(item->Tradeskills);
if (id == "cr")
stat = uint32(item->CR);
if (id == "dr")
stat = uint32(item->DR);
if (id == "pr")
stat = uint32(item->PR);
if (id == "mr")
stat = uint32(item->MR);
if (id == "fr")
stat = uint32(item->FR);
if (id == "astr")
stat = uint32(item->AStr);
if (id == "asta")
stat = uint32(item->ASta);
if (id == "aagi")
stat = uint32(item->AAgi);
if (id == "adex")
stat = uint32(item->ADex);
if (id == "acha")
stat = uint32(item->ACha);
if (id == "aint")
stat = uint32(item->AInt);
if (id == "awis")
stat = uint32(item->AWis);
if (id == "hp")
stat = uint32(item->HP);
if (id == "mana")
stat = uint32(item->Mana);
if (id == "ac")
stat = uint32(item->AC);
if (id == "deity")
stat = uint32(item->Deity);
if (id == "skillmodvalue")
stat = uint32(item->SkillModValue);
if (id == "skillmodtype")
stat = uint32(item->SkillModType);
if (id == "banedmgrace")
stat = uint32(item->BaneDmgRace);
if (id == "banedmgamt")
stat = uint32(item->BaneDmgAmt);
if (id == "banedmgbody")
stat = uint32(item->BaneDmgBody);
if (id == "magic")
stat = uint32(item->Magic);
if (id == "casttime_")
stat = uint32(item->CastTime_);
if (id == "reqlevel")
stat = uint32(item->ReqLevel);
if (id == "bardtype")
stat = uint32(item->BardType);
if (id == "bardvalue")
stat = uint32(item->BardValue);
if (id == "light")
stat = uint32(item->Light);
if (id == "delay")
stat = uint32(item->Delay);
if (id == "reclevel")
stat = uint32(item->RecLevel);
if (id == "recskill")
stat = uint32(item->RecSkill);
if (id == "elemdmgtype")
stat = uint32(item->ElemDmgType);
if (id == "elemdmgamt")
stat = uint32(item->ElemDmgAmt);
if (id == "range")
stat = uint32(item->Range);
if (id == "damage")
stat = uint32(item->Damage);
if (id == "color")
stat = uint32(item->Color);
if (id == "classes")
stat = uint32(item->Classes);
if (id == "races")
stat = uint32(item->Races);
if (id == "maxcharges")
stat = uint32(item->MaxCharges);
if (id == "itemtype")
stat = uint32(item->ItemType);
if (id == "material")
stat = uint32(item->Material);
if (id == "casttime")
stat = uint32(item->CastTime);
if (id == "elitematerial")
stat = uint32(item->EliteMaterial);
if (id == "procrate")
stat = uint32(item->ProcRate);
if (id == "combateffects")
stat = uint32(item->CombatEffects);
if (id == "shielding")
stat = uint32(item->Shielding);
if (id == "stunresist")
stat = uint32(item->StunResist);
if (id == "strikethrough")
stat = uint32(item->StrikeThrough);
if (id == "extradmgskill")
stat = uint32(item->ExtraDmgSkill);
if (id == "extradmgamt")
stat = uint32(item->ExtraDmgAmt);
if (id == "spellshield")
stat = uint32(item->SpellShield);
if (id == "avoidance")
stat = uint32(item->Avoidance);
if (id == "accuracy")
stat = uint32(item->Accuracy);
if (id == "charmfileid")
stat = uint32(item->CharmFileID);
if (id == "factionmod1")
stat = uint32(item->FactionMod1);
if (id == "factionmod2")
stat = uint32(item->FactionMod2);
if (id == "factionmod3")
stat = uint32(item->FactionMod3);
if (id == "factionmod4")
stat = uint32(item->FactionMod4);
if (id == "factionamt1")
stat = uint32(item->FactionAmt1);
if (id == "factionamt2")
stat = uint32(item->FactionAmt2);
if (id == "factionamt3")
stat = uint32(item->FactionAmt3);
if (id == "factionamt4")
stat = uint32(item->FactionAmt4);
if (id == "augtype")
stat = uint32(item->AugType);
if (id == "ldontheme")
stat = uint32(item->LDoNTheme);
if (id == "ldonprice")
stat = uint32(item->LDoNPrice);
if (id == "ldonsold")
stat = uint32(item->LDoNSold);
if (id == "banedmgraceamt")
stat = uint32(item->BaneDmgRaceAmt);
if (id == "augrestrict")
stat = uint32(item->AugRestrict);
if (id == "endur")
stat = uint32(item->Endur);
if (id == "dotshielding")
stat = uint32(item->DotShielding);
if (id == "attack")
stat = uint32(item->Attack);
if (id == "regen")
stat = uint32(item->Regen);
if (id == "manaregen")
stat = uint32(item->ManaRegen);
if (id == "enduranceregen")
stat = uint32(item->EnduranceRegen);
if (id == "haste")
stat = uint32(item->Haste);
if (id == "damageshield")
stat = uint32(item->DamageShield);
if (id == "recastdelay")
stat = uint32(item->RecastDelay);
if (id == "recasttype")
stat = uint32(item->RecastType);
if (id == "augdistiller")
stat = uint32(item->AugDistiller);
if (id == "attuneable")
stat = uint32(item->Attuneable);
if (id == "nopet")
stat = uint32(item->NoPet);
if (id == "potionbelt")
stat = uint32(item->PotionBelt);
if (id == "stackable")
stat = uint32(item->Stackable);
if (id == "notransfer")
stat = uint32(item->NoTransfer);
if (id == "questitemflag")
stat = uint32(item->QuestItemFlag);
if (id == "stacksize")
stat = uint32(item->StackSize);
if (id == "potionbeltslots")
stat = uint32(item->PotionBeltSlots);
if (id == "book")
stat = uint32(item->Book);
if (id == "booktype")
stat = uint32(item->BookType);
if (id == "svcorruption")
stat = uint32(item->SVCorruption);
if (id == "purity")
stat = uint32(item->Purity);
if (id == "backstabdmg")
stat = uint32(item->BackstabDmg);
if (id == "dsmitigation")
stat = uint32(item->DSMitigation);
if (id == "heroicstr")
stat = uint32(item->HeroicStr);
if (id == "heroicint")
stat = uint32(item->HeroicInt);
if (id == "heroicwis")
stat = uint32(item->HeroicWis);
if (id == "heroicagi")
stat = uint32(item->HeroicAgi);
if (id == "heroicdex")
stat = uint32(item->HeroicDex);
if (id == "heroicsta")
stat = uint32(item->HeroicSta);
if (id == "heroiccha")
stat = uint32(item->HeroicCha);
if (id == "heroicmr")
stat = uint32(item->HeroicMR);
if (id == "heroicfr")
stat = uint32(item->HeroicFR);
if (id == "heroiccr")
stat = uint32(item->HeroicCR);
if (id == "heroicdr")
stat = uint32(item->HeroicDR);
if (id == "heroicpr")
stat = uint32(item->HeroicPR);
if (id == "heroicsvcorrup")
stat = uint32(item->HeroicSVCorrup);
if (id == "healamt")
stat = uint32(item->HealAmt);
if (id == "spelldmg")
stat = uint32(item->SpellDmg);
if (id == "ldonsellbackrate")
stat = uint32(item->LDoNSellBackRate);
if (id == "scriptfileid")
stat = uint32(item->ScriptFileID);
if (id == "expendablearrow")
stat = uint32(item->ExpendableArrow);
if (id == "clairvoyance")
stat = uint32(item->Clairvoyance);
// Begin Effects
if (id == "clickeffect")
stat = uint32(item->Click.Effect);
if (id == "clicktype")
stat = uint32(item->Click.Type);
if (id == "clicklevel")
stat = uint32(item->Click.Level);
if (id == "clicklevel2")
stat = uint32(item->Click.Level2);
if (id == "proceffect")
stat = uint32(item->Proc.Effect);
if (id == "proctype")
stat = uint32(item->Proc.Type);
if (id == "proclevel")
stat = uint32(item->Proc.Level);
if (id == "proclevel2")
stat = uint32(item->Proc.Level2);
if (id == "worneffect")
stat = uint32(item->Worn.Effect);
if (id == "worntype")
stat = uint32(item->Worn.Type);
if (id == "wornlevel")
stat = uint32(item->Worn.Level);
if (id == "wornlevel2")
stat = uint32(item->Worn.Level2);
if (id == "focuseffect")
stat = uint32(item->Focus.Effect);
if (id == "focustype")
stat = uint32(item->Focus.Type);
if (id == "focuslevel")
stat = uint32(item->Focus.Level);
if (id == "focuslevel2")
stat = uint32(item->Focus.Level2);
if (id == "scrolleffect")
stat = uint32(item->Scroll.Effect);
if (id == "scrolltype")
stat = uint32(item->Scroll.Type);
if (id == "scrolllevel")
stat = uint32(item->Scroll.Level);
if (id == "scrolllevel2")
stat = uint32(item->Scroll.Level2);
safe_delete(inst);
return stat;
}
|
 |
|
 |

03-14-2014, 06:39 PM
|
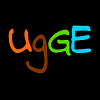 |
Developer
|
|
Join Date: Apr 2012
Location: North Carolina
Posts: 2,815
|
|
I'm assuming that $client->SummonItem() calls the actual server code and is not script-defined elsewhere.
This is the server code: https://github.com/EQEmu/Server/blob...ntory.cpp#L203
I added the lore checks some time ago..but, have not incorporated any validity checks, as yet, into the existing code..so, for now, you'll
have to manually check them in scripts.
(I will add validation in the rework..probably with a status override as well.)
Btw, thanks for bringing this up! I just found a memory leak that I created!
</facepalm>
EDIT: I took a closer look at the code..so, no memory leak. But, it could still use some tweaking.
__________________
Uleat of Bertoxxulous
Compilin' Dirty
|

03-16-2014, 07:41 PM
|
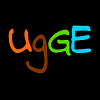 |
Developer
|
|
Join Date: Apr 2012
Location: North Carolina
Posts: 2,815
|
|
I have a working patch for Client::SummonItem() to check wear/restriction validity.
It needs to be tested more thoroughly before committing..but, it puts checks (with rules) in for augment validation.
This is in the source code..so, nothing needs to be done to #summonitem or $client->SummonItem() - except maybe a handler
for item creation failure (change from void to bool on the return type.)
__________________
Uleat of Bertoxxulous
Compilin' Dirty
|
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 11:32 PM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |