|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
Quests::Q&A This is the quest support section |
 |
|
 |

07-02-2021, 05:08 PM
|
Fire Beetle
|
|
Join Date: Jul 2021
Posts: 1
|
|
No Duplicate Loot
Lets say I want a raid mob to drop 3 unique pieces of loot from a single lootdrop,
the current lootdrop functionality doesn't seem to have a way for me to do that.
So instead I'm looking at adding loot through a Perl script instead.
Here's what I've got so far:
Code:
sub EVENT_SPAWN{
my @item_PHARA = (1628, 1845, 1848, 2377, 10845, 10851, 10912, 10913, 24648); #loot list
my $itemlist = @item_PHARA;
my $item1 = $item_PHARA[plugin::RandomRange(0,$itemlist)];
quest::addloot($item1, 0, 0);
@item_PHARA2 = grep {$_ != $item1 } @item_PHARA; #remove first loot from loot array, then draw another item
my $itemlist2 = @item_PHARA2;
my $item2 = $item_PHARA2[plugin::RandomRange(0,$itemlist2)];
quest::addloot($item2, 0, 0);
@item_PHARA3 = grep {$_ != $item2 } @item_PHARA2; #remove second loot from loot array, then draw another item
my $itemlist3 = @item_PHARA3;
my $item3 = $item_PHARA3[plugin::RandomRange(0,$itemlist3)];
quest::addloot($item3, 0, 0);
}
It seems to mostly work, only problem is about 40% of the time only 2 loots are added instead of 3.
No clue if I'm using the grep function correctly, I tried googling how to remove a value from an array and that seemed to be a potential solution.
Thanks for looking
|
 |
|
 |
 |
|
 |

07-02-2021, 06:32 PM
|
Banned
|
|
Join Date: Mar 2021
Posts: 14
|
|
Quote:
Originally Posted by klausfelix
Lets say I want a raid mob to drop 3 unique pieces of loot from a single lootdrop,
the current lootdrop functionality doesn't seem to have a way for me to do that.
So instead I'm looking at adding loot through a Perl script instead.
Here's what I've got so far:
Code:
sub EVENT_SPAWN{
my @item_PHARA = (1628, 1845, 1848, 2377, 10845, 10851, 10912, 10913, 24648); #loot list
my $itemlist = @item_PHARA;
my $item1 = $item_PHARA[plugin::RandomRange(0,$itemlist)];
quest::addloot($item1, 0, 0);
@item_PHARA2 = grep {$_ != $item1 } @item_PHARA; #remove first loot from loot array, then draw another item
my $itemlist2 = @item_PHARA2;
my $item2 = $item_PHARA2[plugin::RandomRange(0,$itemlist2)];
quest::addloot($item2, 0, 0);
@item_PHARA3 = grep {$_ != $item2 } @item_PHARA2; #remove second loot from loot array, then draw another item
my $itemlist3 = @item_PHARA3;
my $item3 = $item_PHARA3[plugin::RandomRange(0,$itemlist3)];
quest::addloot($item3, 0, 0);
}
It seems to mostly work, only problem is about 40% of the time only 2 loots are added instead of 3.
No clue if I'm using the grep function correctly, I tried googling how to remove a value from an array and that seemed to be a potential solution.
Thanks for looking
|
I see what you're trying to do but you're making it a bit more complicated than it needs to be.
There is a plugin that comes with the base install called "AddLoot" that does what you're trying to do already (minus the "unique" functionality).
Code:
sub EVENT_SPAWN {
my @items = (1, 2, 3, 4);
plugin::AddLoot(1, 1, @items);
}
^ brief example of how it works. If you want it be for sure unique you could either make 3 separate arrays. Or you could edit the plugin to return the ID of the item it chooses and then use one of these to edit your array accordingly.
|
 |
|
 |

07-02-2021, 07:05 PM
|
Sarnak
|
|
Join Date: May 2007
Posts: 47
|
|
Or just split the loottables up into 3 sets?
|

07-03-2021, 10:36 AM
|
 |
Discordant
|
|
Join Date: Jan 2007
Posts: 443
|
|
Yeah, I guess I'm confused why you don't just use multiple lootdrops...
|

07-03-2021, 07:06 PM
|
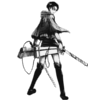 |
Developer
|
|
Join Date: Dec 2012
Posts: 515
|
|
Code:
sub EVENT_SPAWN {
my @item_PHARA = (1628, 1845, 1848, 2377, 10845, 10851, 10912, 10913, 24648); #loot list
#my $itemlist = @item_PHARA;
my $item1 = splice(@item_PHARA, plugin::RandomRange(0,$#item_PHARA), 1);
my $item2 = splice(@item_PHARA, plugin::RandomRange(0,$#item_PHARA), 1);
my $item3 = splice(@item_PHARA, plugin::RandomRange(0,$#item_PHARA), 1);
quest::addloot($item1, 0, 0);
quest::addloot($item2, 0, 0);
quest::addloot($item3, 0, 0);
}
Maybe try that, it should splice the array by removing 1 element and placing it into the $item1 variable... then 2 and 3 ect...
or go even further and do this...
Code:
sub EVENT_SPAWN {
my @item_PHARA = (1001, 1002, 1003, 1004, 1005, 1006, 1007, 1008, 1009); #loot list
for $item (1 .. 3) { #drop 3 items...
my $item_drop = splice(@item_PHARA, plugin::RandomRange(0,$#item_PHARA), 1);
quest::addloot($item_drop, 0, 0);
}
}
Last edited by NatedogEZ; 07-03-2021 at 07:21 PM..
|
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 07:26 PM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |